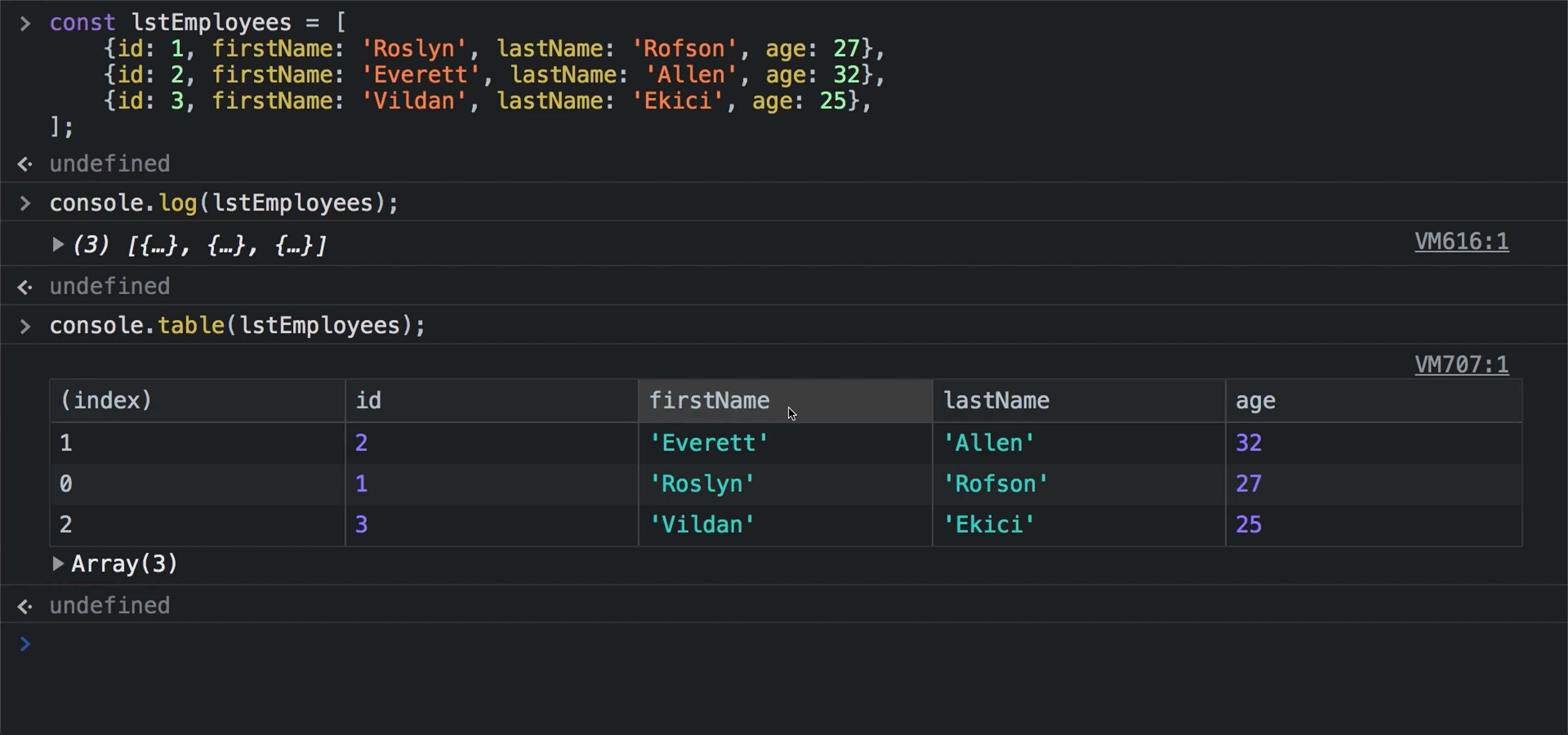
Use console.table for better visualization of arrays and objects
Last Updated: May 5, 2022
Introduction
Have you ever found a need to visualize array/object as table so that you can
- quickly see value of a particular property for all the items in an array
- sort array items by value of a particular property
- see values of group of properties together
- quickly see values of nested objects
Such need often arises when we are getting data from backend using some undocumented REST API and we want to get overview of what different kind of data different properties can contain across all the items.
We can do all the things mentioned above using console.table()
method.
First, let's understand how to use console.table()
method.
How to use console.table() method
Similar to how console.log()
method logs output on the console, console.table()
method also logs output on the console but in table form and each column of the table is sortable.
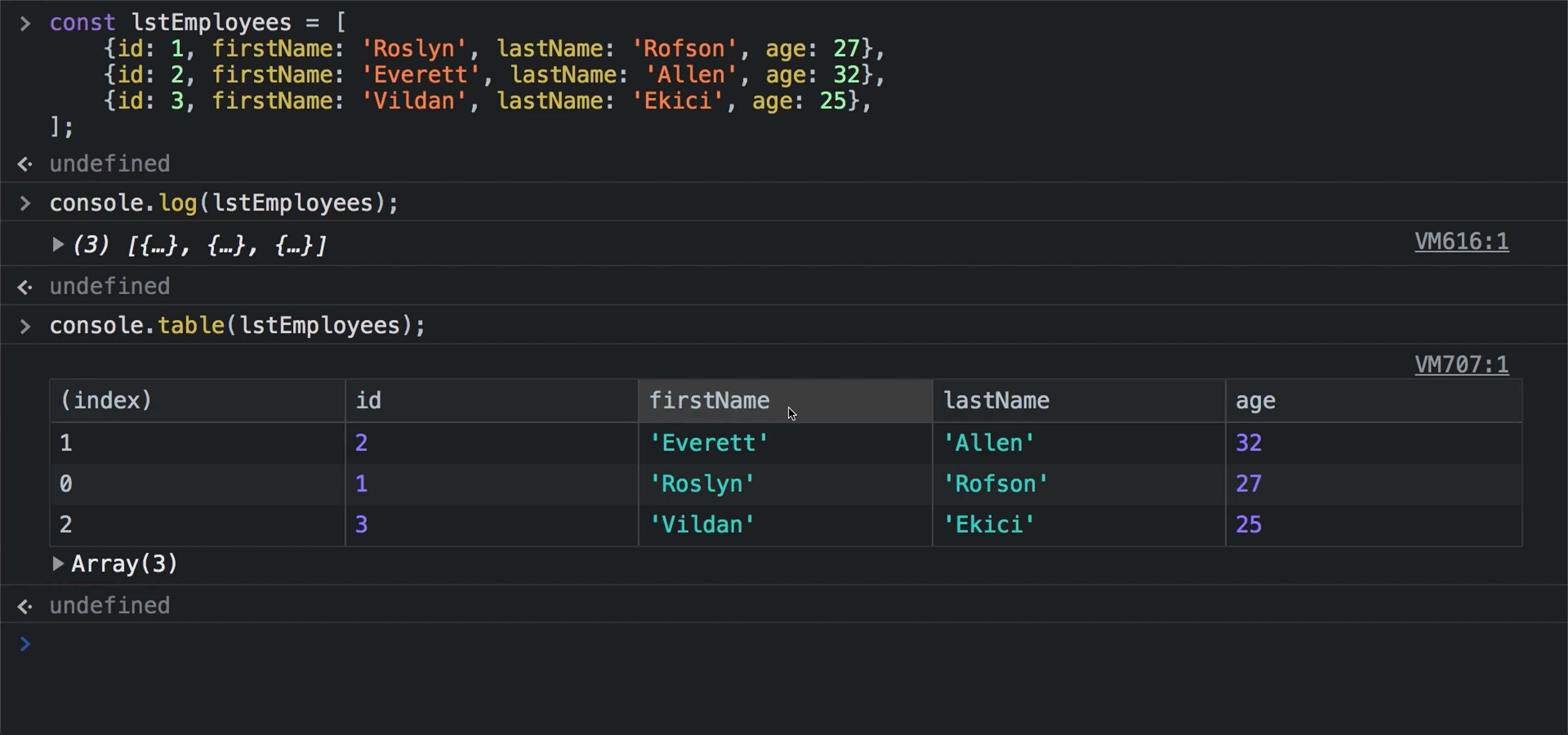
Syntax of console.table method
console.table(data, columns)
method accepts two parameters:
data
can be array or object.columns
is an optional parameter. It is an array of property names which we want to see in table. More about how to use this parameter is covered later in this post.
Let's explore all use cases of console.table()
method.
Use console.table for array of objects
We can just pass array of objects in first parameter of console.table()
method to see the array of objects as table.
const lstEmployees = [ { id: 1, firstName: 'Roslyn', lastName: 'Rofson', age: 27 }, { id: 2, firstName: 'Everett', lastName: 'Allen', age: 32 }, { id: 3, firstName: 'Vildan', lastName: 'Ekici', age: 25 }, ]; console.table(lstEmployees);
Output:
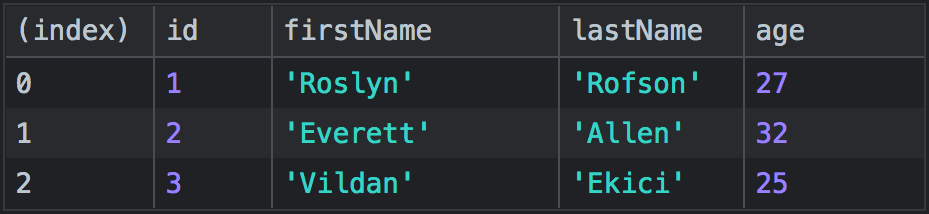
As shown in output, first column always shows index of the array when we pass array as data to console.table()
method.
Show only some properties in console.table output
By default console.table()
method shows all the properties of the object of data array.
So, if we have so many properties in an object, the table does not look clean.
To, solve that problem, we can pass list of property names in second parameter of the console.table()
method.
const lstEmployees = [ { id: 1, firstName: 'Roslyn', lastName: 'Rofson', age: 27 }, { id: 2, firstName: 'Everett', lastName: 'Allen', age: 32 }, { id: 3, firstName: 'Vildan', lastName: 'Ekici', age: 25 }, ]; console.table(lstEmployees, ['id', 'firstName']);
Output:
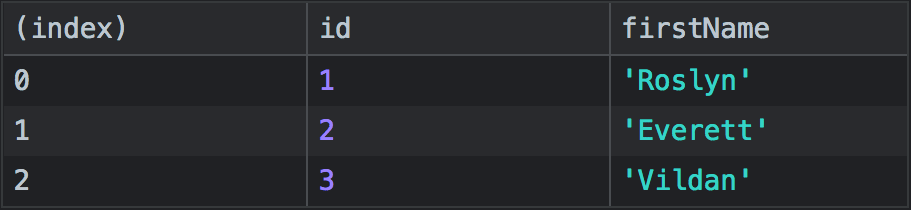
As shown in output, the table shows only index, id and firstName columns.
Note that we cannot remove index
columns from the display. It will always shown when we are pass array as data to console.table()
method.
Use console.table method for two-dimensional array
console.table()
method can be useful to visualize two-dimensional arrays such as graph data, CSV data etc. as table.
const csvText = `id,firstName,lastName,age 1,Roslyn,Rofson,27 2,Everett,Allen,32 3,Vildan,Ekici,25`; /* Sample code to convert csv data to array */ // const csvData = csvText.split('\n').map(item => item.split(',')) const csvData = [ ['id', 'firstName', 'lastName', 'age'], [ 1, 'Roslyn', 'Rofson', 27 ], [ 2, 'Everett', 'Allen', 32 ], [ 3, 'Vildan', 'Ekici', 25 ], ]; console.table(csvData);
Output:

As shown in output, in case of two-dimensional array, rows and columns of the table show index. Also, we cannot set which columns to see by passing second argument to console.table()
method.
Use console.table method for object
We can pass an object as data to console.table()
method. In this case first column shows property names and second column shows values of those properties.
const employee = { id: 1, firstName: 'Roslyn', lastName: 'Rofson', age: 27 }; console.table(employee);
Output:

Note that in this case we cannot pass columns
parameter to console.table()
method.
Use console.table method for nested object
When we pass nested objects to console.table()
method,
- it shows name of enumerable properties of root object in first column
- creates separate column for each enumerable properties of nested object
- shows values of nested object properties as separate columns in each row
const employeesByRole = { 'manager': { id: 2, firstName: 'Everett', lastName: 'Allen', age: 32 }, 'dba':{ id: 1, firstName: 'Roslyn', lastName: 'Rolfson', age: 27 }, 'developer': { id: 3, firstName: 'Vildan', lastName: 'Ekici', age: 25 } }; console.table(employeesByRole);
Output:

Show only some properties of nested object
We can pass names of nested object property as columns
in console.table()
method to show only those properties in table.
const employeesByRole = { 'manager': { id: 2, firstName: 'Everett', lastName: 'Allen', age: 32 }, 'dba':{ id: 1, firstName: 'Roslyn', lastName: 'Rolfson', age: 27 }, 'developer': { id: 3, firstName: 'Vildan', lastName: 'Ekici', age: 25 } }; console.table(employeesByRole, ['id', 'firstName']);
Output:

That's how we can use console.table()
method in various use cases to visualize array or object data better as table.
Do you have any question related to this post? Just Ask Me on Twitter